Introduction
If you’re preparing for a PHP developer interview, understanding common PHP interview questions can help you stand out. Whether you’re a beginner or an experienced developer, this guide covers essential questions that interviewers often ask.
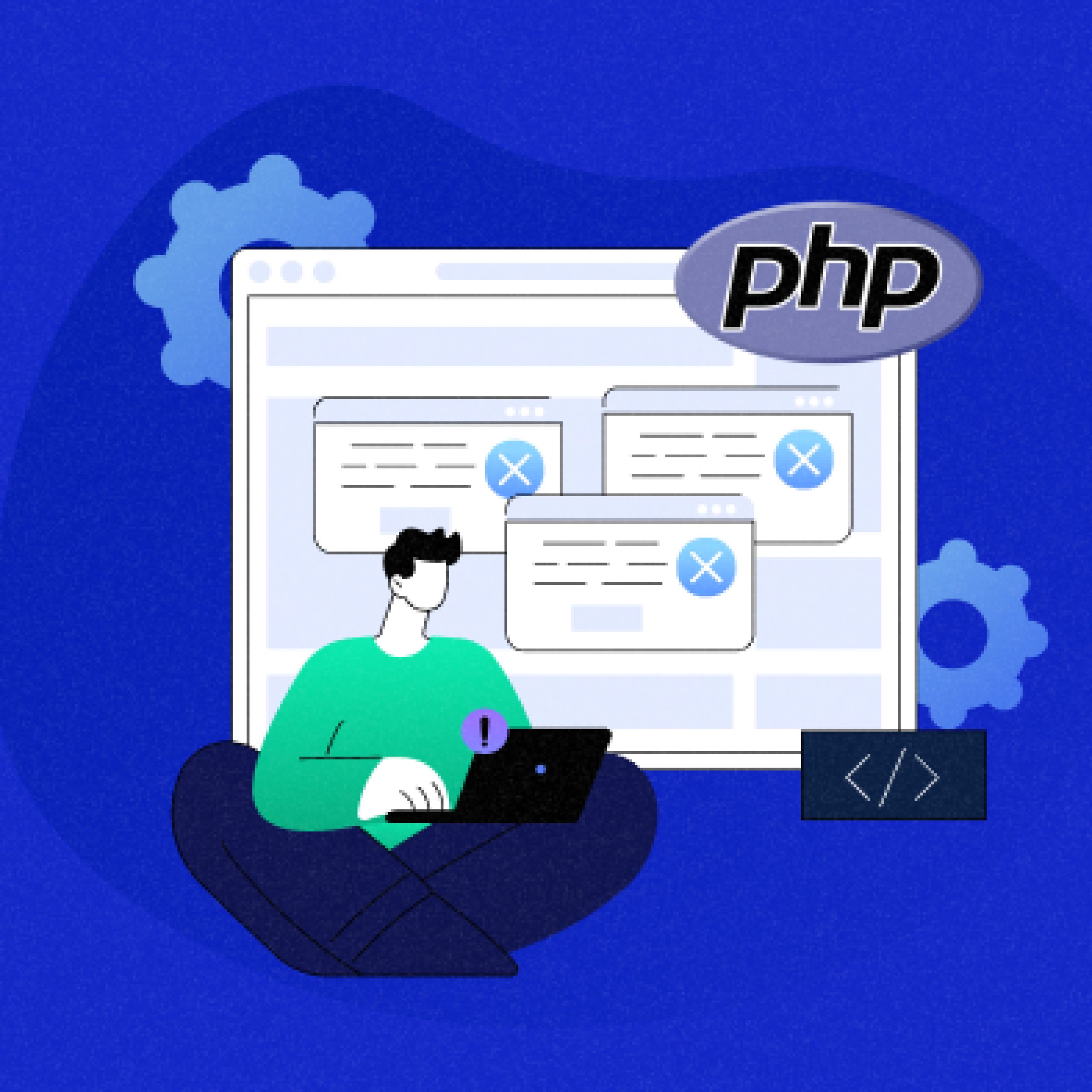
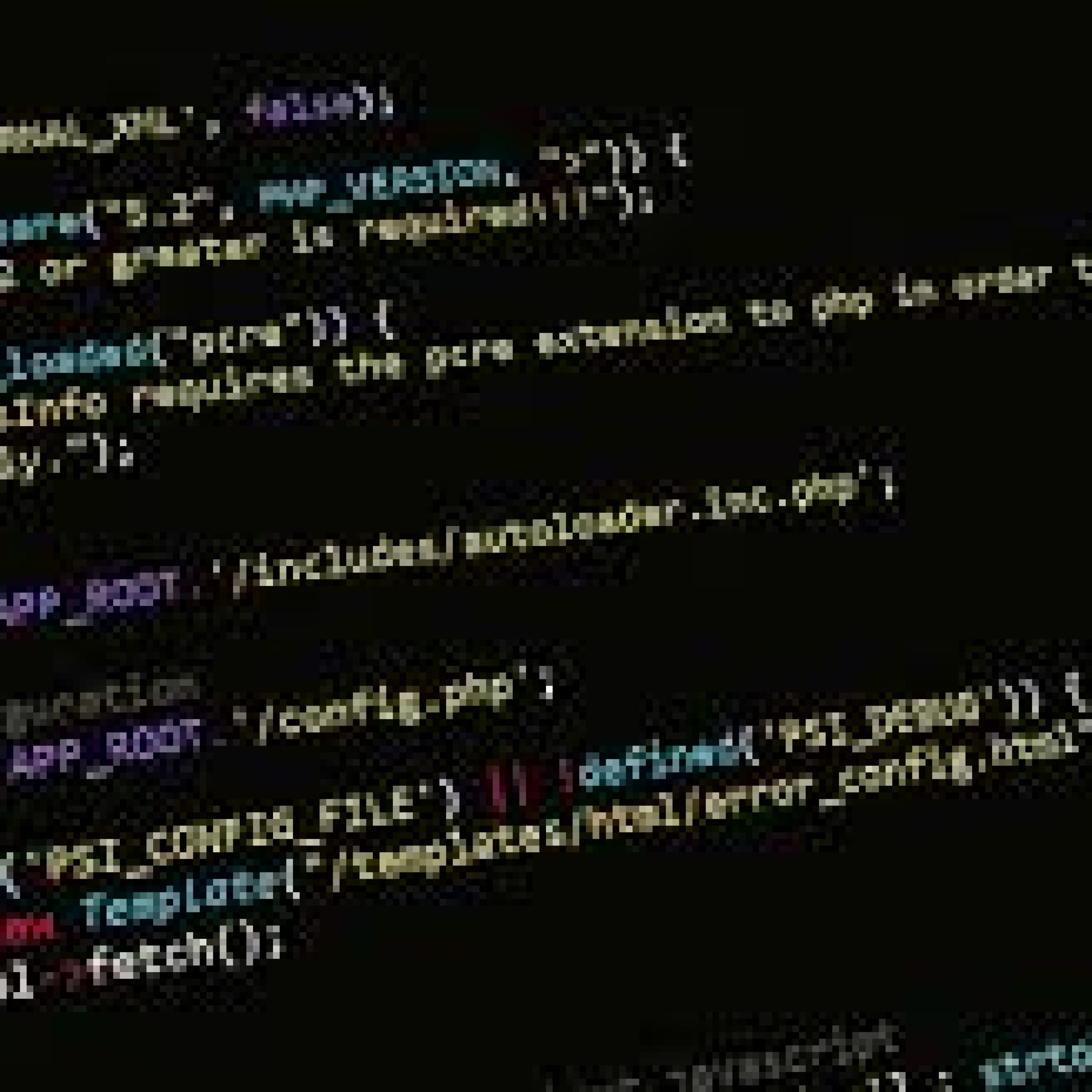
Basic PHP Interview Questions
1. What is PHP?
PHP (Hypertext Preprocessor) is a widely-used, open-source scripting language suited for web development. It runs on the server side and helps generate dynamic web pages.
2. What are the key features of PHP?
- Open-source and free to use
- Cross-platform compatibility
- Supports databases like MySQL, PostgreSQL, and SQLite
- Simple syntax, easy to integrate with HTML
- Supports Object-Oriented Programming (OOP)
3. What is the difference between echo and print in PHP?
Both echo and print are used to output data, but:
- echo can take multiple parameters and is slightly faster.
- print returns a value (1), so it can be used in expressions.
4. How do you declare variables in PHP?
PHP variables start with a $ sign:
- $name = “John Doe”;
- $age = 30;
- Variables in PHP are case-sensitive.
Intermediate PHP Interview Questions
5. What are super global variables in PHP?
Super global variables are built-in PHP arrays that provide information from server, session, and user input.
Examples include:
- $_GET – Retrieves data from URL parameters
- $_POST – Collects form data
- $_SESSION – Stores session variables
- $_COOKIE – Handles user cookies
- $_SERVER – Provides server information
6. What is the difference between include and require?
- include – Includes a file, but if the file is missing, it will throw a warning and continue execution.
- require – Includes a file, but if the file is missing, it will throw a fatal error and halt execution.
7. How does PHP handle errors?
PHP offers various error handling methods:
- error_reporting(E_ALL); – Displays all errors.
- try-catch blocks – Used for exception handling.
- set_error_handler() – Custom error handlers.
8. What is the use of explode() and implode() in PHP?
- explode(delimiter, string) – Splits a string into an array.
- implode(delimiter, array) – Joins array elements into a string.
Example:
- $str = “apple,banana,orange”;
- $fruits = explode(“,”, $str);
Advanced PHP Interview Questions
9. What is a PHP session?
A session allows data storage across multiple pages.
- session_start();
- $_SESSION[‘username’] = “JohnDoe”;
Sessions are stored on the server and persist until the browser is closed or manually destroyed.
10. What is Composer in PHP?
- Composer is a dependency manager for PHP. It helps install and manage external libraries.
- composer require monolog/monolog
11. How does PHP interact with databases?
- PHP connects to databases using MySQLi or PDO.
- $conn = new mysqli(“localhost”, “username”, “password”, “database”);
- PDO is more secure and recommended for database interactions.
Best Practices for PHP Development
- Always sanitize user input to prevent SQL injection.
- Use prepared statements when dealing with databases.
- Enable error reporting during development and disable it in production.
- Follow MVC architecture for scalable applications.
Conclusion
Preparing for a PHP job interview requires a strong grasp of both basic and advanced concepts. By reviewing these PHP interview questions, you’ll be ready to showcase your knowledge and coding skills confidently.
For more PHP resources, explore our PHP development tutorials and stay updated with the latest trends.